Rust on STM32
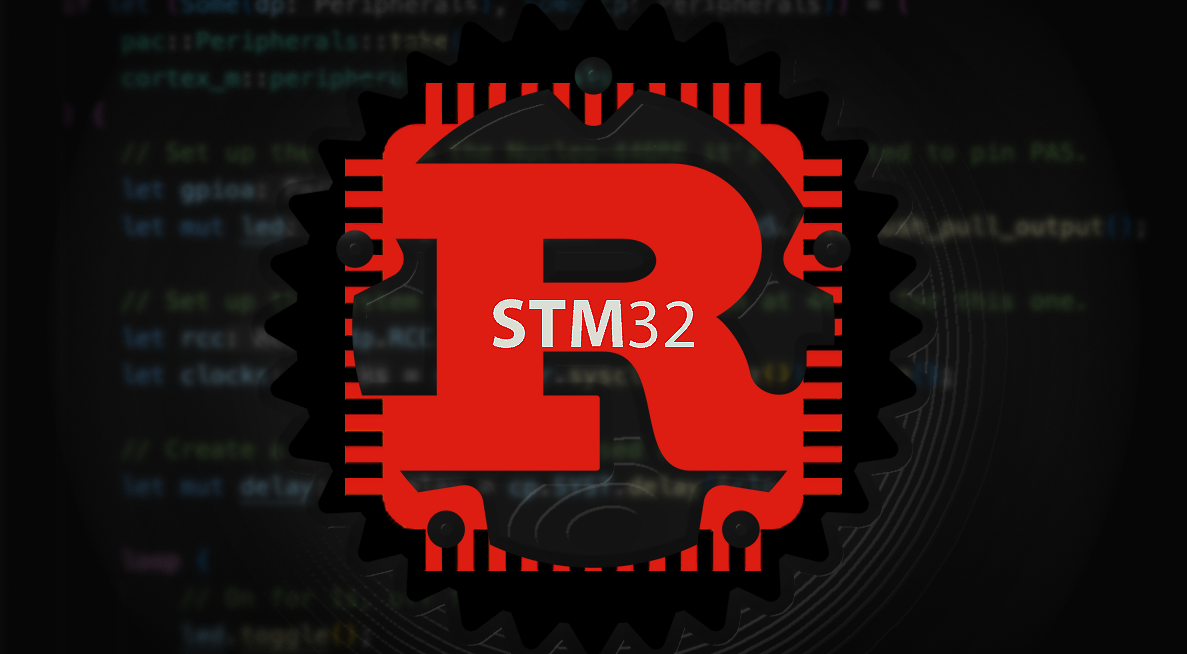
Hi all, in this short tutorial I want to show you how I get a blinking LED on an NUCLEO-F411RE which uses the STM32F411RE chip. I got some trouble to getting all the necessary steps together, so I would like to collect them in this blog post. I compile on macOS, but the steps should be similar to Linux.
Installing Prerequisites
First you have to install the target for the Cortex M4 with FPU support.
rustup target add thumbv7em-none-eabihf
also you have to install the probe-run Cargo that uploads the program onto the board.
cargo install probe-run
Getting started
first we initialize a new project by navigating to a new location and execute
cargo init
Download the stm32f4xx-hal library from GitHub and replace the content of the main.rs file with the content of the examples/delay-syst-blinky.rs
Next, add the dependencies to the Cargo.toml file
[dependencies]
embedded-hal = "0.2"
nb = "1"
cortex-m = "0.7"
cortex-m-rt = "0.7"
# Panic behaviour, see https://crates.io/keywords/panic-impl for alternatives
panic-halt = "0.2"
[dependencies.stm32f4xx-hal]
version = "0.17.1"
features = ["stm32f411"] # replace the model of your microcontroller here
# and add other required features
Note that if you have a different board, you should replace it under features.
a full list of which boards are available can be found on the table found in the README of the stm32f4xx-hal library.
For the next step, we copy the .cargo folder and the memory.x into our main project folder. We now have to edit the memory.x to match our STM32 Version.
MEMORY
{
/* NOTE K = KiBi = 1024 bytes */
FLASH : ORIGIN = 0x08000000, LENGTH = 512K
RAM : ORIGIN = 0x20000000, LENGTH = 128K
}
Run Our Program
Now we can try to run our program by executing
cargo run
after you reset the board, you should see the LED Blinking SUCCESS
cargo add defmt
and then try cargo rung again
If you are using VS Code, rust-analyzer shows falsely an error
you can fix this by adding
{
"rust-analyzer.cargo.target": "thumbv7em-none-eabihf",
"rust-analyzer.checkOnSave.allTargets": false
}
to the .vscode/settings.json file.
Final
you can find the Documentation for the HAL library HERE
I hope the tutorial helps with getting started on Programming STM32 in the Rust programming language. If you have any additions, feel free to write them in the comments.